Source: Read Barcodes from Image, PDF, and Word with Python | by Alexander Stock | Medium
Jul 17, 2024
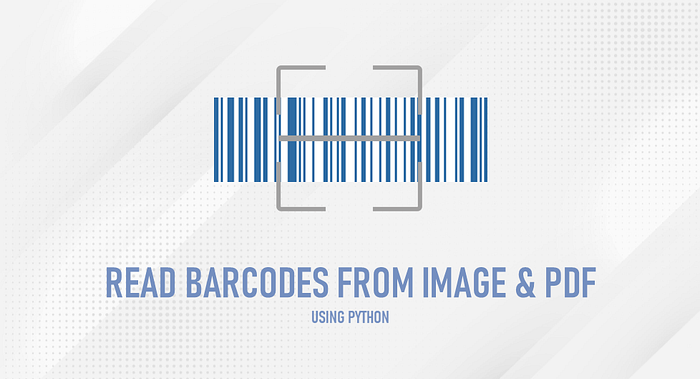
Being able to efficiently extract and interpret barcode data from digital documents like images, PDF files, and Word documents can provide significant benefits for businesses and organizations. It streamlines data entry, improves inventory accuracy, and enables more effective automation of various business processes.
In this blog post, I’ll walk you through the process of reading barcodes from these types of digital sources using Python.
- Read Barcodes from Images in Python
- Read Barcodes from PDF in Python
- Read Barcodes from Word in Python
Python Library for Reading Barcodes
To read barcodes from image files, I use the Spire.Barcode for Python library. This library supports the detection and scanning of over 38 common barcode types, including Code 39, Code 128, EAN-8, PDF417, QR Code, and Data Matrix, from images in any orientation.
The library can be installed from PyPI using the following pip command.
pip install Spire.Barcode
Note: Some barcode types are only supported when a license is applied. To unlock all possibilities of the library, you can get a free trail license from the vendor.
Read Barcodes from Images in Python
Spire.Barcode for Python provides the BarcodeScanner
class, which is responsible for recognizing barcodes in images. This class provides the following methods:
ScanOneFile()
: Used to scan an image file that contains a single barcode.ScanFile()
: Used to scan an image file that may contain multiple barcodes.
The following code snippet showcases the recognition of barcodes from image files using Spire.Barcode.
from spire.barcode import *
# Apply license key
License.SetLicenseKey("license key")
# Scan an image file that contains one barcode
result = BarcodeScanner.ScanOneFile("C:\\Users\\Administrator\\Desktop\\MyImage\\barcode_one.jpg")
# Scan an image file that contain multiple barcodes
results = BarcodeScanner.ScanFile("C:\\Users\\Administrator\\Desktop\\MyImage\\barcode_two.jpg")
# Print the results
print(result)
print(results)
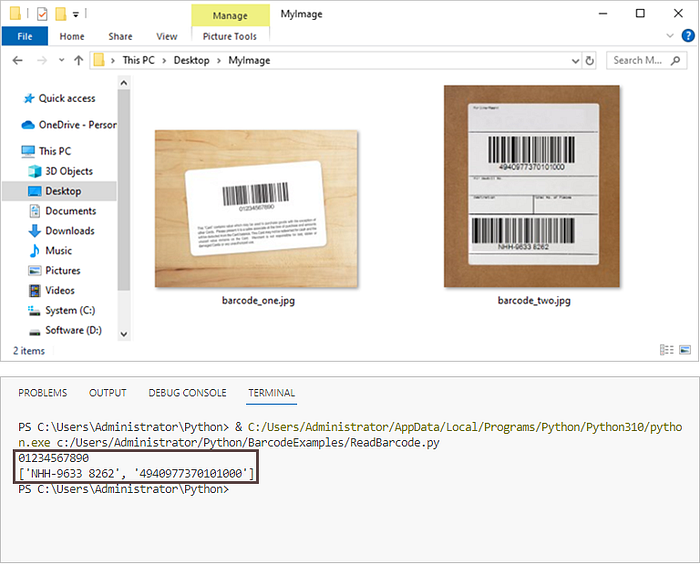
Read Barcodes from PDF in Python
To read barcodes from PDF, an extra library called Spire.PDF for Python is required. And it is responsible for convert a PDF page into an image file. Then, you’ll be able to read barcodes from the image file using Spire.Barcode.
Spire.PDF library can be install PyPI as well.
pip install Spire.PDF
This code shows you how to scan barcodes from a PDF page using Spire.PDF and Spire.Barcode.
from spire.barcode import *
from spire.barcode import License as barcodeLicense
from spire.pdf import *
# Apply license key
barcodeLicense.SetLicenseKey("license key")
# Create a PdfDocument object
doc = PdfDocument()
# Load a PDF file
doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Barcode.pdf")
# Convert the first page to image stream
stream = doc.SaveAsImage(0, PdfImageType.Bitmap)
# Save stream to a PNG image
stream.Save("ToPNG.png")
# Scan barcodes from the image
results = BarcodeScanner.ScanFile("ToPNG.png")
# Print results
print(results)
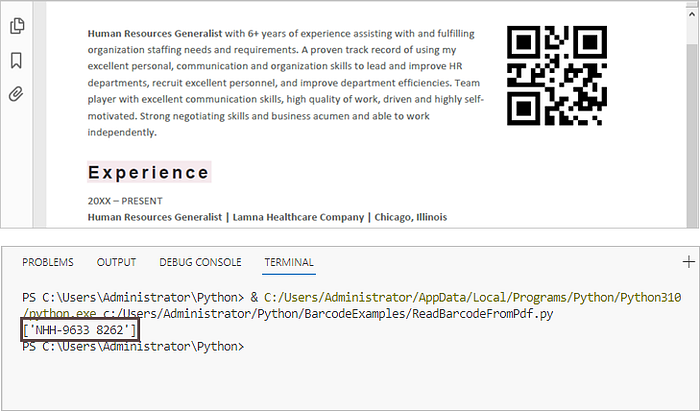
Read Barcodes from Word in Python
In order to read barcodes from Word, we need first to convert Word into image files or image streams using Spire.Doc for Python. This library can be installed through the command:
pip install Spire.Doc
The following code example demonstrates how to convert a Word page into an image file and then extract barcode information from it.
from spire.barcode import *
from spire.barcode import License as barcodeLicense
from spire.doc import *
# Apply license key
barcodeLicense.SetLicenseKey("license key")
# Create a Document object
doc = Document()
# Load a sample Word document
doc.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Barcode.docx");
# Convert the first page to image
stream = doc.SaveImageToStreams(0, ImageType.Bitmap)
# Save the bitmap to a PNG file
with open('ToImage.png','wb') as imageFile:
imageFile.write(stream.ToArray())
# Scan barcode from the image
resutls = BarcodeScanner.ScanFile("ToImage.png")
# Print results
print(resutls)
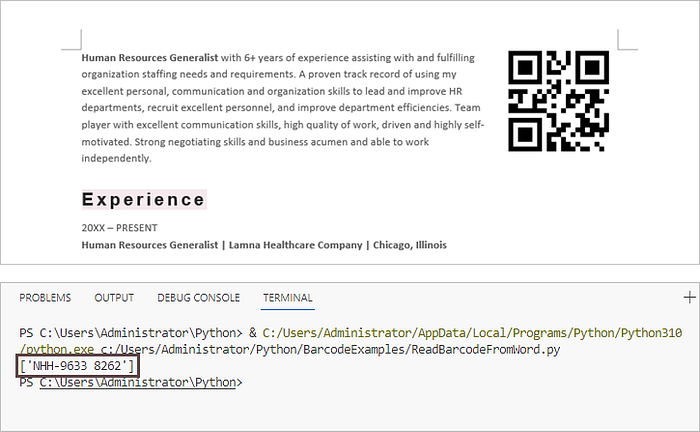
Conclusion
In this article, we’ve explored the methods for reading barcodes from image files, PDF documents, and Word documents using the Spire.Barcode, Spire.PDF, and Spire.Doc libraries.
The Spire.PDF and Spire.Doc libraries are used to convert PDF and Word into images, while the Spire.Barcode library is utilized to recognize and extract barcode information from those images. Alternatively, you can also use other software or tools to convert the digital documents to images before applying barcode recognition techniques.